
刽子手规则
在继续之前,让我们刷新一下刽子手游戏的规则。
- Hangman 是一款快速简单的两人游戏。
- 在第一轮中,第一个玩家(“主机”玩家)想到了一个秘密词。
- 然后,“主机”玩家画出与秘密单词中字母数量相等的破折号。 他还填写了一些字母作为第二个玩家的主要提示。
- 现在,第二个玩家必须通过一次猜一个字母来找到整个秘密单词。
- 如果密语中包含猜出的字母,则“主机”玩家将该字母填入密语的所有相应空白处。 否则,“宿主”玩家会画出刽子手身体的一个元素。 每一次错误的猜测都会使第二名玩家离失败更近一步。
- 如果第二个玩家在“主人”玩家有机会画出整个刽子手之前猜到了这个秘密单词,那么他就赢了。
- “主队”球员和对方球员的角色在下一场比赛中互换。

让我们编码吧!
这个项目包括一些重要的概念,比如对象指针数组、友元函数、继承等。这段代码对初学者很友好,但是,在编码之前确保你理解每个概念(特别是如果你是初学者) .
步骤 0:
首先添加所需的 c++ 标头。
#include<iostream>
#include<fstream>
#include<string.h>
#include<conio.h>
using namespace std;
步骤1:
让我们通过创建一个名为“Game”的新类来开始构建实际游戏。
- 这个类将作为“Hangman”类的父类。
- 您可以稍后添加另一个游戏作为此“游戏”类的子项。
- 我们将在“Game”类中添加一个名为“get_password”的方法。
class Game
{
private:
public:
int get_password()
{
}
};
第2步:
在这一步中,我们将添加“get_password()”方法以及所有相关组件。
- ‘PASSWORD’字符串将存储启动游戏所需的密码。 您可以根据需要更改其值。
- “pass_count”将用于限制错误密码尝试的次数。
- “pass”字符串将存储输入的密码,该密码将与“PASSWORD”字符串进行比较。
class Game
{
private:
string PASSWORD = "HangMan";
char ch;
string pass ="";
int pass_count=3;
public:
int get_password()
{
cout<<"\n\n==============================WELCOME TO THE CONSOLE===========================\n\n";
cout<<"\nYou Need To Enter the Password to play the game ! ";
while(pass_count!=0)
{
pass = "";
cout<<"\n\nPassword : ";
ch = _getch();
while(ch != 13)
{
pass.push_back(ch);
cout << '*';
ch = _getch();
}
if(pass == PASSWORD)
{
return 1;
}
else
{
pass_count--;
cout << "\nWrong Password!!\n";
cout<<pass_count<<" Chances Remaining !!";
}
}
return 0;
}
};
第 3 步:
此外,让我们编写一个将在游戏开始时调用的函数。
- 此功能将标志着游戏的开始。
- 它将显示刽子手的规则。
void begin_HangMan()
{
cout<<"\n\t\t\t\tH H MM MM\n\t\t\t\tH H M M M M\n\t\t\t\tHHHHHHH M M M\n\t\t\t\tH H M M\n\t\t\t\tH H M M";
cout<<"\n\nInstructions : \n1. This Game Required Minimum Two Players.";
cout<<"\n2. One player has to enter a word and the other players needs to identify the that word using one letter at a time before he is hanged.";
cout<<"\n3. The words will be saved in a file and we can view it later.";
//cout<<"\n4. ";
}
第4步:
接下来,让我们构建“HangMan”类
- 此类将为“HangMan”游戏的多个实例提供蓝图。
- 我们还将使用一个名为“机会”的朋友功能来继续游戏。
class HangMan : public Game
{
private:
int marks=0, len=0, player=1, z=0;
char ch; // str[100]; //arr[50] = {'a','e','i','o','u'};
string str;
public:
HangMan(int p)
{
player = p%2 + 1;
cout<<"\nPlayer "<<player<<" : ";
cout<<"\nEnter a Word (Only small characters): ";
ch = _getch();
while(ch != 13)
{
str.push_back(ch);
cout << '*';
ch = _getch();
}
cout<<"\n\n\n\n\n\n\n";
}
int friend chance(HangMan *a);
};
第 5 步:
现在让我们构建“机会”方法。
- 此方法将运行“刽子手”游戏背后的逻辑。
- 它将接受一个“HangMan”对象作为函数参数。
- 该方法将调用另一个方法“draw”,该方法负责绘制“hangman”的当前状态。
int chance(HangMan *a)
{
int i=0, j=0, len=0, flag1=0, flag2, x=1, z=5, count=10;
char c, letter, arr[40]={'a','e','i','o','u'};
len = strlen(a->str);
z = strlen(arr);
while(x!=7)
{
count=0;
for(i=0; i<len; i++)
{
flag1=0;
for(j=0; j<z; j++)
{
if(a->str[i]==arr[j])
{
cout<<"\t"<<arr[j]<<"\t";
flag1=1;
}
}
if(flag1==0)
{
cout<<"\t"<<"_"<<"\t";
count++;
}
}
if(count==0)
{
cout<<"\n\nCONGRATULATIONS - you have found the RIGHT WORD\n";
return 10;
}
cout<<"\n\nEnter a character : ";
cin>>letter;
arr[z] = letter;
z++;
flag2 = 0;
for(i=0;i<len;i++)
{
if(letter == a->str[i])
{
cout<<"\nThis Letter is Present !\n";
flag2 = 1;
}
}
if(flag2!=1)
{
x = draw(x);
}
}
return 0;
}
第 6 步:
为了显示‘hangman’的状态,将使用‘draw’方法。
整数将作为参数传递。
根据整数的值,将决定“刽子手”的这种状态。
此方法将返回一个整数,指示刽子手的下一个状态。
int draw(int l)
{
switch(l)
{
cout<<"\n===============================Current Position==================================\n ";
case 0: cout<<"\n\n\t\t 6 more chances remaining ";
//cout<<"\n\n\t\t\t O";
return 1;
break;
case 1: cout<<"\n\n\t\t 5 more chances remaining ";
cout<<"\n\n\t\t\t O\n\n\n";
return 2;
break;
case 2: cout<<"\n\n\t\t 4 more chances remaining ";
cout<<"\n\n\t\t\t O\n\t\t\t /\n\n\n";
return 3;
break;
case 3: cout<<"\n\n\t\t 3 more chances remaining ";
cout<<"\n\n\t\t\t O\n\t\t\t / \\\n\n\n";
return 4;
break;
case 4: cout<<"\n\n\t\t 2 more chances remaining ";
cout<<"\n\n\t\t\t O\n\t\t\t / | \\\n\t\t\t |\n\t\t\t\n\n\n";
return 5;
break;
case 5: cout<<"\n\n\t\t 1 more chances remaining ";
cout<<"\n\n\t\t\t O\n\t\t\t / | \\\n\t\t\t |\n\t\t\t / \n\t\t\t / \n\n\n";
return 6;
break;
case 6: cout<<"\n\n\t\t OOPS You are DEAD !!";
cout<<"\n\n\t\t\t O\n\t\t\t / | \\\n\t\t\t |\n\t\t\t / \\\n\t\t\t / \\";
return 7;
break;
}
}
第 7 步:
最后,让我们写出 main 函数。
- 我们将在这里创建一个游戏对象。
- 根据输入的密码,“刽子手”游戏将开始或结束。
- 我们将创建一个指向新创建对象的对象指针数组。
- 每一轮都将使用一个新对象。
- 最后,“delete”关键字将用于销毁所有创建的对象。
int main()
{
char c1,c2;
int i=0, j=0, marks=0, pass=0, choice;
Game o;
pass = o.get_password();
if(pass == 1)
{
cout << "\nAccess granted! Lets PLAY!! \n";
}
else
{
cout<<"\n================================OOPS! Too Many Attemps! ==================================================";
return 0;
}
HangMan *ptr[40];
begin_HangMan();
while(1)
{
cout<<"\n\n\nDo you want to Enter a new Word? (y/n) : ";
cin>>c1;
if (c1=='y' || c1=='Y')
{
ptr[i] = new HangMan(i);
marks = chance(ptr[i]);
//ptr[i]->setMarks(marks1);
i++;
}
else
{
break;
}
}
for(j=0;j<i;j++)
{
delete ptr[j];
}
cout<<"\n\nThank You For Trying Out HangMan.";
cout<<"\nHope You Enjoyed!\n\n";
}
输出




潜在的改进
虽然可以进行许多细微的调整以使这款游戏变得更好,但以下是更大的潜在改进,可以使游戏更加令人印象深刻:
1.添加积分系统:
- 我们可以为每个玩家添加一个标记系统。
- 玩家的分数可以基于他/她猜测秘密单词的尝试次数。
- 累积分数可以稍后显示以宣布获胜者。
2.添加额外的游戏
- 我们可以添加新类作为“游戏”类的子类。
- 这些新类可以包含其他游戏。
3.添加界面:
- 可以进行的另一项改进是为玩家添加一个干净的 UI。
- 游戏不仅吸引人,而且对用户友好。
快乐编码
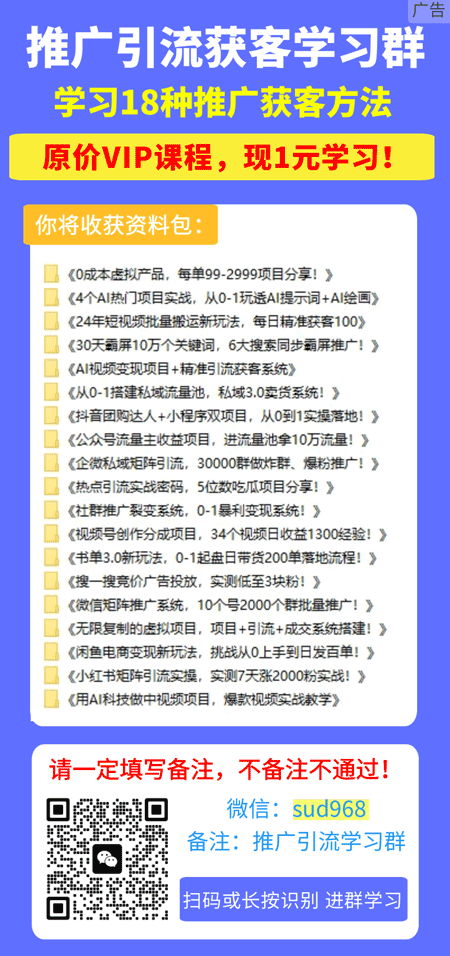
本文内容由互联网用户自发贡献,该文观点仅代表作者本人。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如发现本站有涉嫌抄袭侵权/违法违规的内容, 请发送邮件至 sumchina520@foxmail.com 举报,一经查实,本站将立刻删除。
如若转载,请注明出处:https://www.gooyie.com/6049.html
如若转载,请注明出处:https://www.gooyie.com/6049.html